2D A* Pathfinding System
February, 2024
This 2D A* pathfinding system in Unity features grid-based visualization, animated path rendering, and dynamic obstacle handling, and tile costs. The project demonstrates real-time pathfinding logic with adjustable parameters, offering a practical and visual approach to understanding the algorithm.
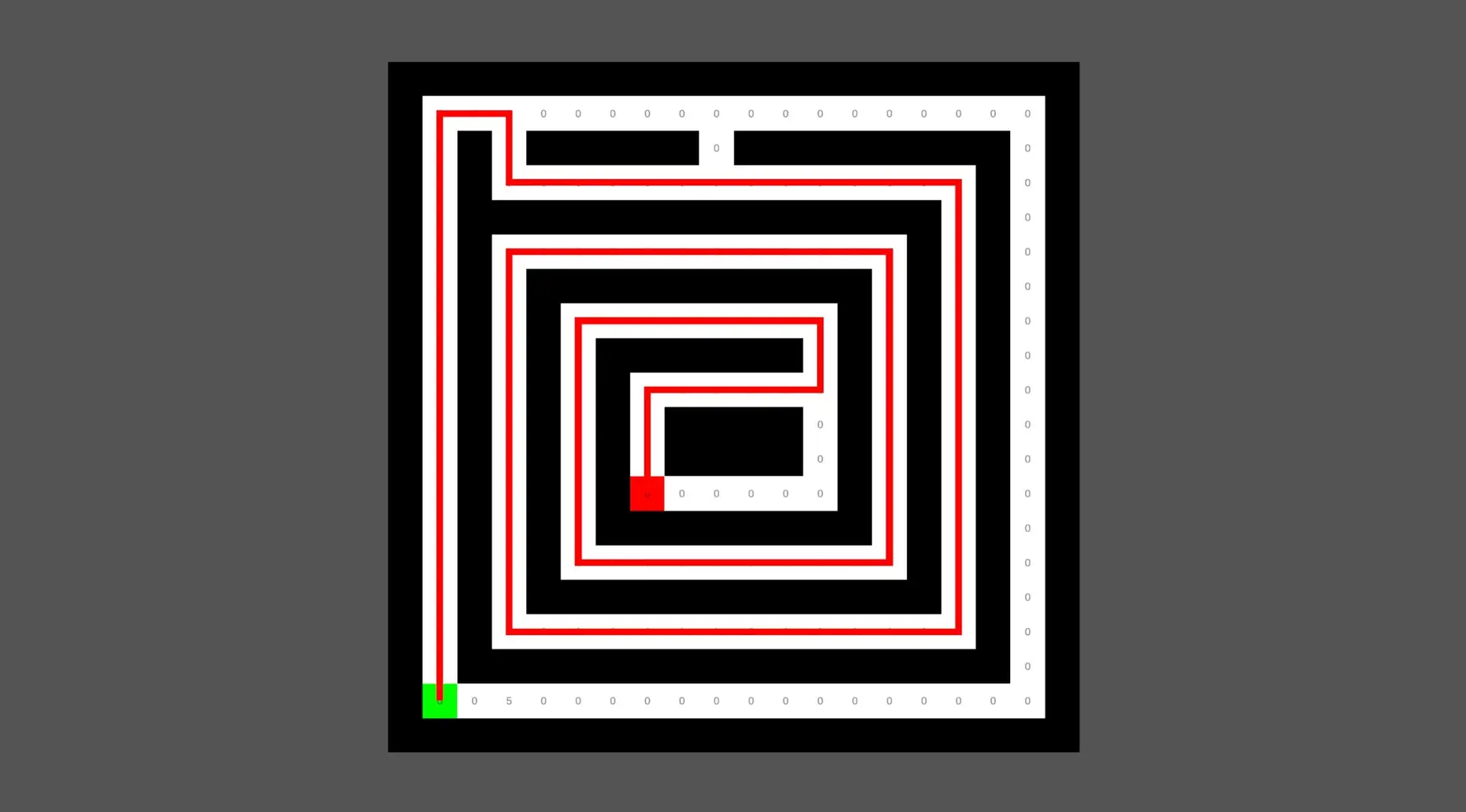
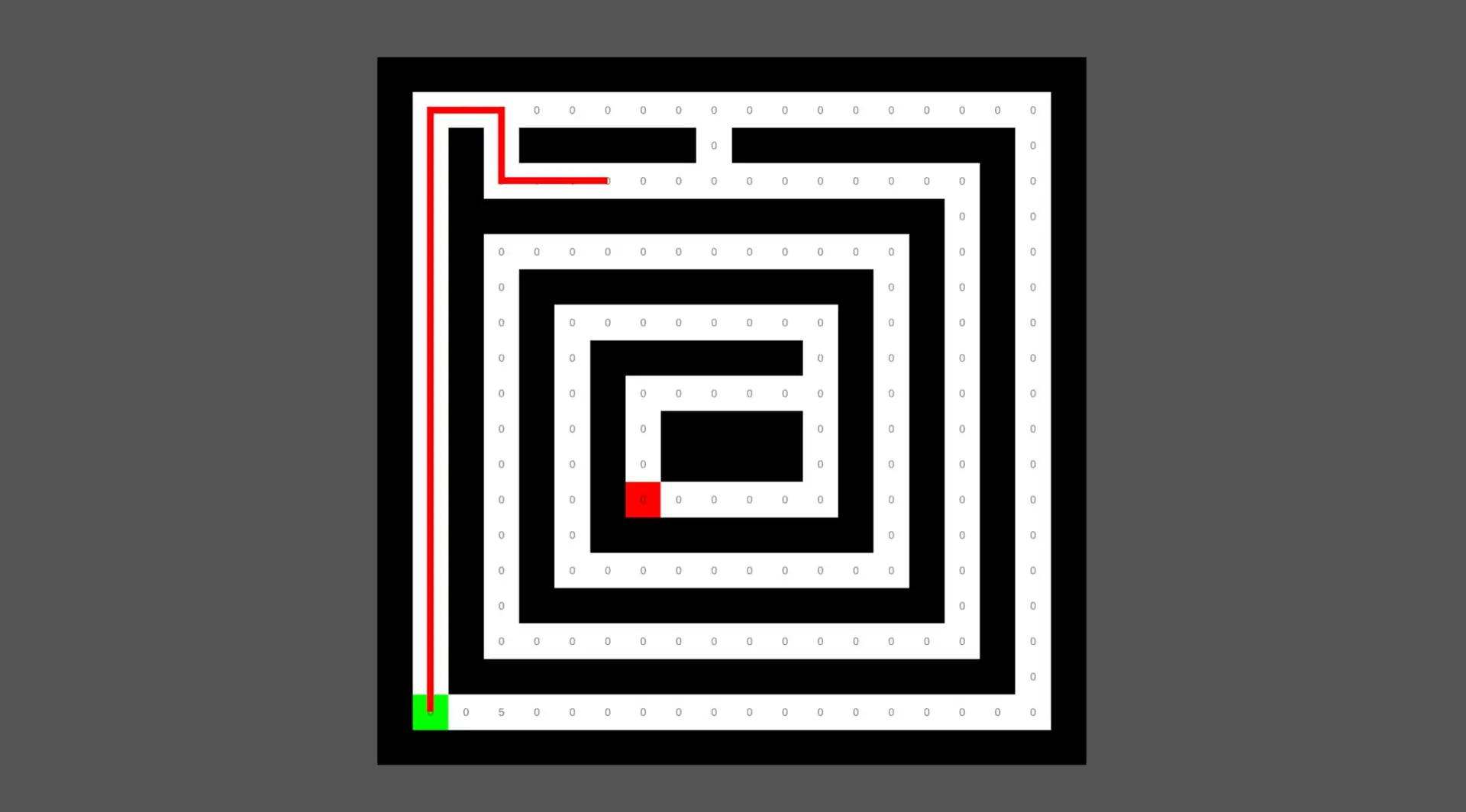
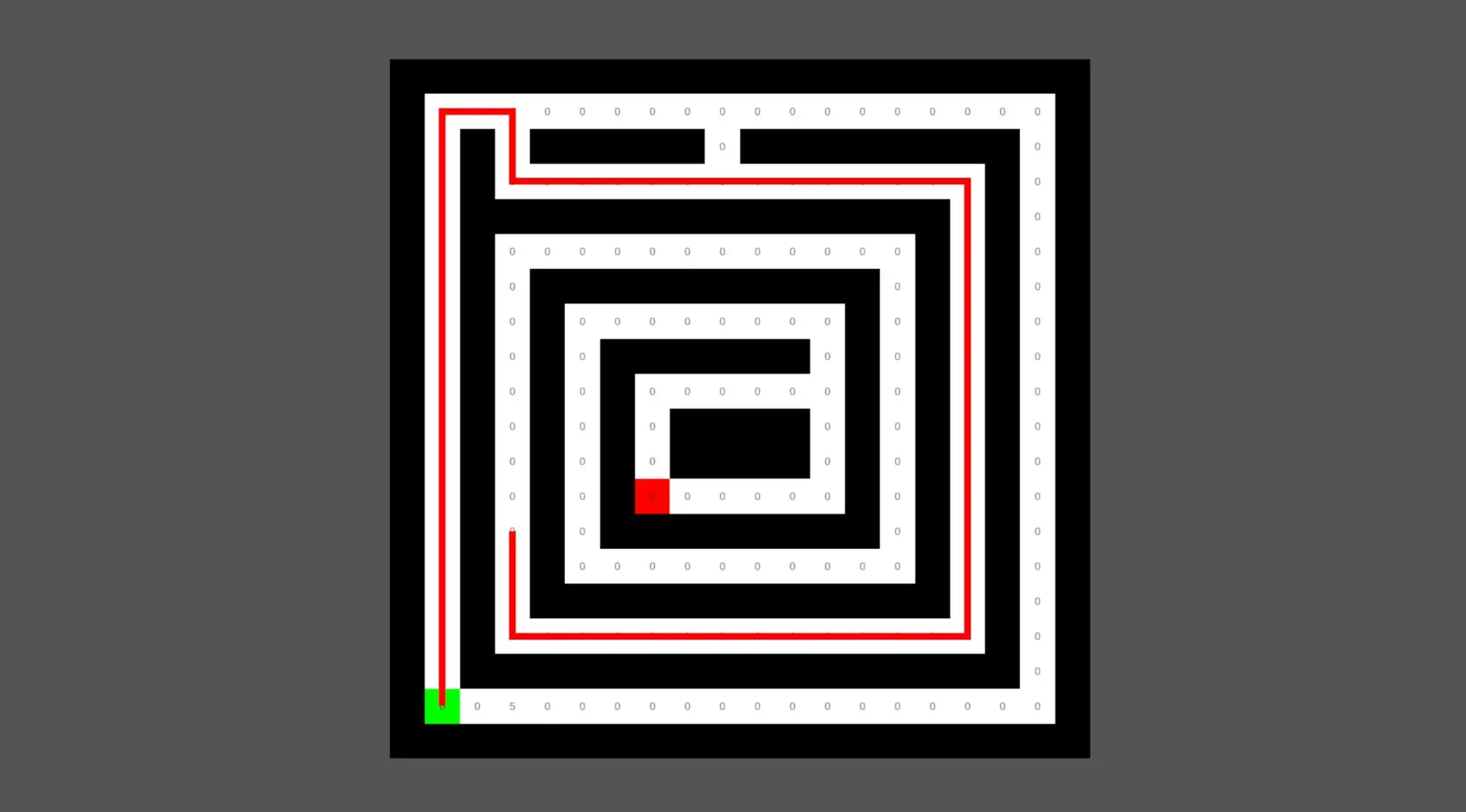
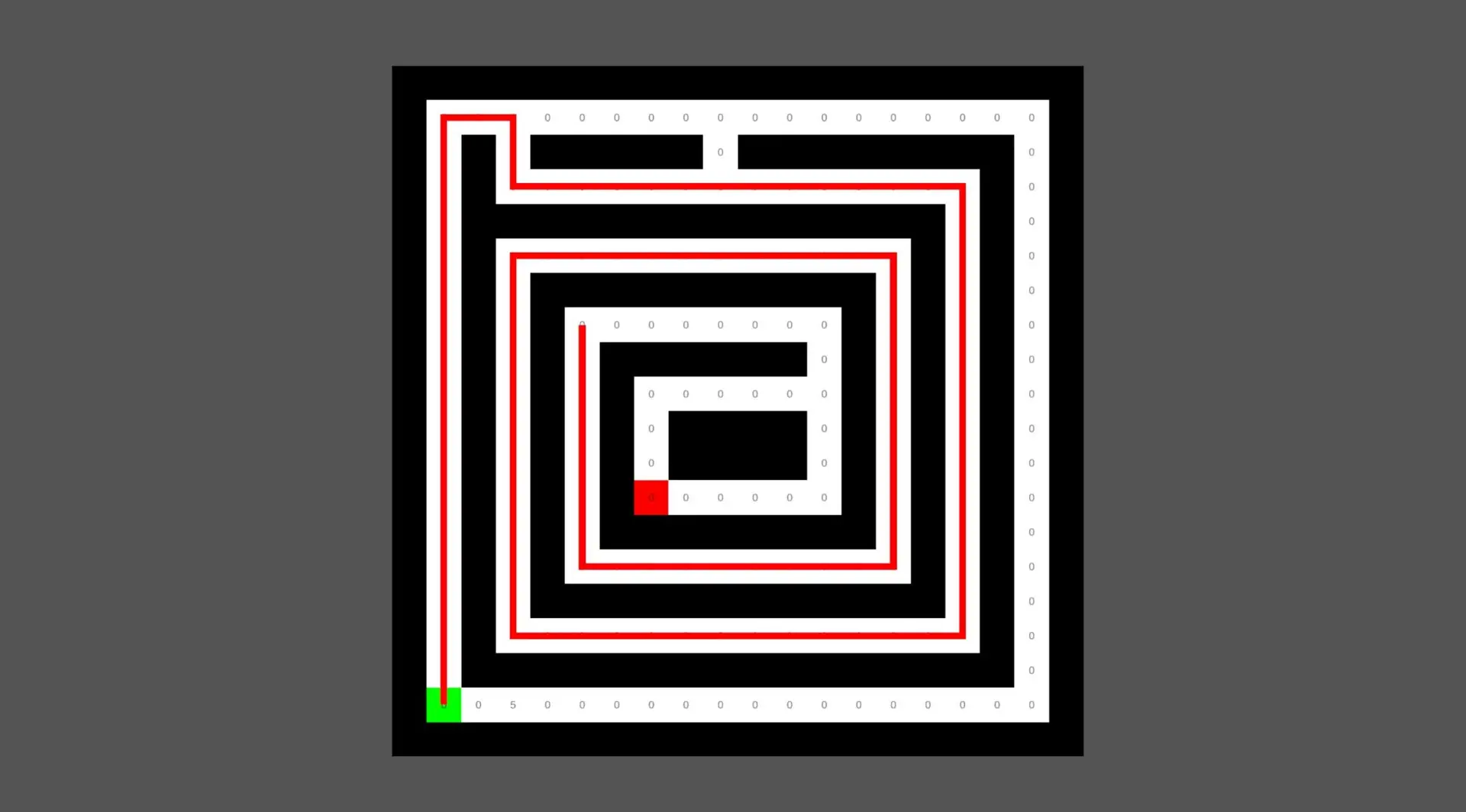
Introduction
- Overview: This project demonstrates a basic 2D implementation of the A* pathfinding algorithm, visualized with a grid-based UI in Unity.
- Motivation: My goal was to gain a deeper understanding of the A* algorithm by implementing it in a system that could visualize its real-time behavior in response to different parameters. This approach allowed me to explore the algorithm from both a coding perspective and a conceptual one, observing how it adapts and responds to various conditions.
Key Features
- A* Pathfinding Algorithm: The system calculates the shortest path between two points while avoiding obstacles. It evaluates possible paths, factoring in movement costs to choose the most efficient route.
- Grid-Based UI: The algorithm operates on a grid where tiles are marked as walkable or non-walkable, with each tile potentially having a unique movement cost.
- Animated Path Visualization: The path is animated by drawing one path point at a time.
- Real-Time Adaptability: The system continuously evaluates changes in the grid, updating paths based on tile states and costs.
Key Challenges & Solutions
1. Writing the A* Algorithm in C#
- Challenge: Translating A* pseudo-code into Unity C# required a deep understanding of its flow, variables, and logic to ensure accurate pathfinding.
- Solution: Broke down the A* algorithm into logical steps and implemented it iteratively, using debugging to verify correct path calculations at each stage. This method ensured a robust, bug-free system capable of dynamically responding to grid changes.
2. Path Visualization
- Challenge: Creating dynamic, animated path visualization.
- Solution: Used Unity’s LineRenderer with a coroutine to animate the path-drawing process, iteratively rendering the path one vector point at a time for a step-by-step effect.
Future Development
Potential enhancements include:
- 3D Adaptation: Expanding this implementation to handle 3D environments.
- Advanced Obstacles: Adding features like moving obstacles or other external variables affecting the pathfinding logic.
- Improved UI: Further refining the grid UI for better visual clarity and aesthetics.